The building blocks of web development
- Kristian Hristov
- Jan 8, 2020
- 4 min read
Updated: Jul 28, 2023
For better or for worse, HTML, CSS and JavaScript are the building blocks of web development. This trio is behind everything we see on the Internet today.
Let’s break it down a bit and see who does what.
HTML is at the bottom of everything
As most of us already know, HTML is the language used to create web pages. These are defined by HTML documents. Our browsers take these documents and render them on screen so we can see the content.
In order for the browser to interpret the document we need to use a common language the browser will understand. In this case HTML is the language we use which is based on tags.
Each tag has a specific purpose to structure the document. For example, if you want to show a title on a document, you would use an h1 tag like this <h1>My Document</h1>. If you want to write a paragraph, you would use a p tag like so:
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam cursus nisi ut facilisis vestibulum. Mauris in lacus vel lectus mattis pharetra. Aenean accumsan ut erat sed condimentum. Etiam laoreet ultricies tincidunt. Fusce in scelerisque nisi. Curabitur ut neque risus. Nulla vitae sagittis ex. Cras ullamcorper velit sed tortor auctor consectetur.</p>
The end result of all that would be this:

Doesn’t look very pretty, does it? Luckily, there’s CSS to the rescue.
CSS to style the document
Remember that HTML is only used to define the content of the document and what the user will get on their screen. Each browser uses a default style for each element in order to see that content, but it is very basic.
In order to make our pages look attractive, we use CSS rules to apply fonts, colors, positions, etc. Think of it as the make up for web pages.
One thing to remember is that while we can have HTML documents without CSS, we can’t have it the other way around.
There are three ways to apply CSS to your HTML documents.
One option is to have the styles defined in each tag.
<h1 style="font-family: sans-serif; font-weight: bolder; color: blue;">My Document</h1>
This is great but it’s not very reusable. It means that every blue heading in your document or whole website will have to have this inline style. The issue is that when you want to make all heading red, you will have to go fixing it one by one.
Inline styles
A possible solution to the above usability issue is to have the styles defined inline in the HTML document. This means you will not use the style attribute inside each tag. Instead you will use a special style tag.
<style type="text/css">
h1 {
font-family: sans-serif;
font-weight: bolder;
color: blue;
}
</style>
You get the same result as above, but now all your headings will have the same styles, and you can control their styles from a single place.
But what happens if you have many pages in your website and all of them have blue headings.
External files for styles
The third and most common way to implement CSS is to have a separate file for all the rules, and then add it to each HTML document.
With this option you can create a single file to hold all of your style rules and then add it to each document. That way you will have a center point to control how your website looks. This solution looks like this:
// site.css file
h1 {
font-family: sans-serif;
font-weight: bolder;
color: blue;
}
// index.html file
<head>
<title>Test document</title>
<link ref="stylesheet" href="./site.css">
</head>
<body>
<h1>My Document</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam cursus nisi ut facilisis vestibulum. Mauris in
lacus vel lectus mattis pharetra. Aenean accumsan ut erat sed condimentum. Etiam laoreet ultricies tincidunt. Fusce
in scelerisque nisi. Curabitur ut neque risus. Nulla vitae sagittis ex. Cras ullamcorper velit sed tortor auctor
consectetur.
</p>
</body>
</html>
Same results as before, but much more reusable.
JavaScript to make things dynamic
HTML and CSS are great, but they are just not enough to meet the expectations of the modern user. The pages we create with HTML and CSS are too static. Users want things to move, get a result when they click a button, etc. For that we use JavaScript.
Just like with CSS, we can add our scripts inline in the document using the script tag, or by linking an external .js document.
// site.js file
function myFunction() {
// do something here
}
// index.html file
<head>
<title>Test document</title>
<link ref="stylesheet" href="./site.css">
<script type="text/javascript" src="./site.js"></script>
</head>
<body>
<h1>My Document</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam cursus nisi ut facilisis vestibulum. Mauris in
lacus vel lectus mattis pharetra. Aenean accumsan ut erat sed condimentum. Etiam laoreet ultricies tincidunt. Fusce
in scelerisque nisi. Curabitur ut neque risus. Nulla vitae sagittis ex. Cras ullamcorper velit sed tortor auctor
consectetur.
</p>
</body>
</html>
We’re not going to review how to make a script as it goes beyond the scope of this article. But be sure we will go into more detail in future releases.
With JavaScript we can turn static websites into complex web applications.
A web application differs from a website in that it can take user inputs and process them somehow and return a result. Think of Facebook or Instagram, where you provide some information and hit Register, the JS code takes your data and passes it along to the server, then takes you to the wall where your can search for friends and create posts, etc.
All that is done transparently to us users, but there’s a big bunch of JavaScript code behind the scenes doing all sorts of witchcrafts.
The web as we know it
Nowadays we can’t imagine life without the internet. We do everything online – shop, talk to people, do business, keep our health record, etc. And while everyone is using the awesome features of their favorite web apps, few of us actually think about what makes the “clock tick”.
Well, it’s this dynamic trio that has taken the internet from the dark ages of static documents to the full scale digital social hive we have today. Hopefully, now you have a better idea of what is behind your browser and which one of these three technologies is in charge of content, presentation and functionality. And now you know what it will take to develop your web application.
Make sure you subscribe to our newsletter to get more content like this in the future.
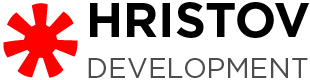
תגובות